Key Classes
Key Classes
This section describes some of the key classes and methods you should know in order to code components and pages for EP Commerce for Adobe Marketing Cloud.
The following diagram shows the key classes of the Adobe Commerce API and Elastic Path
Commerce API implementation. The methods shown in the diagram are only the ones that provide
associations between the key classes. For more details on the Adobe Commerce API, please refer
to their Javadocs.
AEM Commerce API Key Classes
- CommerceServiceFactory - Entry point into the Adobe Commerce API. You don't need to invoke this class directly. You can fetch CommerceService using this pattern: resource.adaptTo(CommerceService.class). AEM will search the resource tree looking for a commerceProvider property and use the search for a matching commerce provider.
- CommerceService - A Service class that handles basic connectivity to the commerce server. Use this class to get a CommerceSession by calling login(SlingHttpServletRequest request, SlingHttpServletResponse response). The Elastic Path implementation will perform the necessary login steps the first time the method is called on a request. Subsequent calls will retrieve the session from the request.
- CommerceSession - The central repository for Adobe Commerce API data. Most of the data required for the components and pages you are building can be retrieved from this class. We recommend consulting the Adobe Javadocs to get an understanding of what is available in this class.
- CommerceSearchProvider - Provides Cortex search functionality. We recommend using service.search(CommerceQuery) on the CommerceService to access search in your components and pages.
The example class sequence diagram below shows how these classes interact to load the Product Details Page:
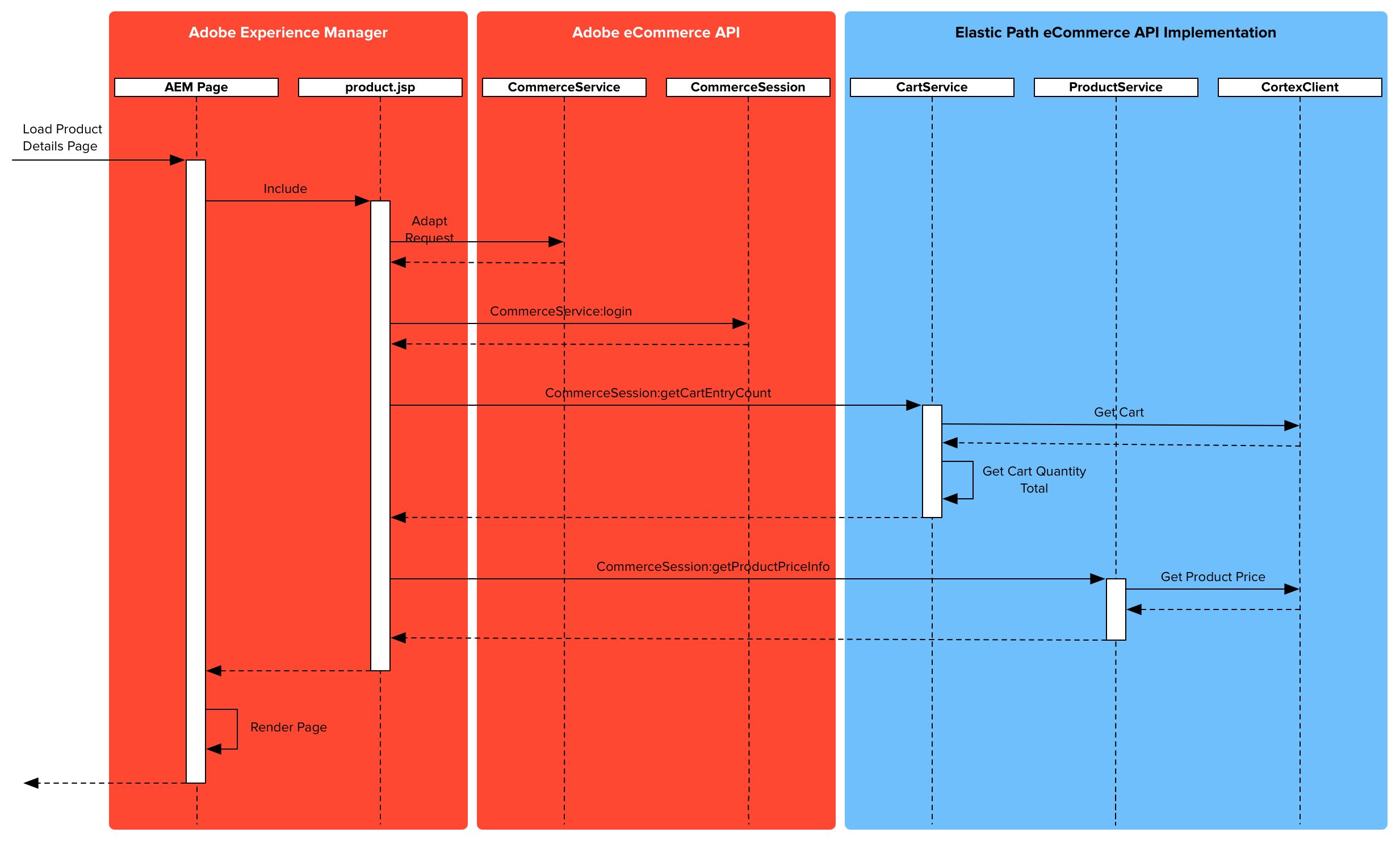
Elastic Path Commerce API Implementation Key Classes
- CortexContext - Encapsulates Cortex's authentication context. To use Cortex resources, you must have a context. Cortex has two allowable roles PUBLIC or REGISTERED.
- AemCortexContextManager - Manages the persistence and retrieval of contexts. Contexts are stored in a browser cookie with some content stored on the user's profile. Storing context data on a user's profile allows Cortex and AEM to associate their users together and validate that they are the same. The out of the box implementation is an OSGi service.
- CortexClientFactory - Provides access to Cortex Java SDK and the CortexClient facade for user-scoped Cortex calls. Most interaction with Cortex will be through a CortexClient instance.
- JAX-RS Client - Provides direct connections to other HTTP services and/or Cortex for cases the do not require a user-scoped connection. This Client service is thread-safe and reusable between requests. The Client can be injected by using the OSGi @Reference annotation. For more information on the JAX-RS services, see the JAX-RS specification.
- CortexSdkServiceFactory - Provides access to services that manage Cortex.
Cortex Java SDK Usage Example
In the class sequence diagram above, the CartService uses Cortex Java SDK to communicate with Cortex. The sample code below shows how Cortex Java SDK is used in the service to get the shopper's cart
Cart
- cart = cortexClient.get(Cart.class);
Methods are omitted from the Cart class for brevity. The example shows how many resources can be zoomed to create the cart used by Adobe Experience Manager. For more details on the annotations available, please refer to the Cortex Java SDK Documentation
- @EntryPointUri({"carts", EntryPointUri.SCOPE, EntryPointUri.DEFAULT})
- @Zoom({
- @RelationPath({"lineitems", "element"}),
- @RelationPath({"lineitems", "element", "item", "price"}),
- @RelationPath({"lineitems", "element", "item", "code"}),
- @RelationPath({"lineitems", "element", "total"}),
- @RelationPath({"total"}),
- @RelationPath({"appliedpromotions", "element"}),
- @RelationPath({"lineitems", "element", "appliedpromotions", "element"}),
- @RelationPath({"discount"})
- })
- public class Cart extends Linkable {
- @JsonPath("$._lineitems[0]._element")
- private List<LineItem> lineItems;
- @JsonPath("$.total-quantity")
...
Read more